PHP Mailer work with AJAX
Basic Mailer
basic php mailer
php<?phphtml form
header('Access-Control-Allow-Origin: *');
header('Content-Type: application/json');
/*
* CONFIGURE EVERYTHING HERE
*/
// an email address that will be in the From field of the email.
$from = isset($_REQUEST['from']) ? $_REQUEST['from'] : 'example@mail.com';
$from = filter_var($from, FILTER_VALIDATE_EMAIL) ? $from : null;
// an email address that will receive the email with the output of the form
$sendTo = 'dimaslanjaka.superuser@blogger.com';
// subject of the email
$subject = 'New message';
// form field names and their translations.
// array variable name => Text to appear in the email
$fields = array('name' => 'Name', 'surname' => 'Surname', 'phone' => 'Phone', 'email' => 'Email', 'message' => 'Message');
// message that will be displayed when everything is OK :)
$okMessage = 'Contact form successfully submitted. Thank you, I will get back to you soon!';
// If something goes wrong, we will display this message.
$errorMessage = 'There was an error while submitting the form. Please try again later';
/*
* LET'S DO THE SENDING
*/
// if you are not debugging and don't need error reporting, turn this off by error_reporting(0);
error_reporting(E_ALL & ~E_NOTICE);
try {
if (count($_POST) == 0) throw new \Exception('Form is empty');
if (!$from) throw new \Exception('Email is invalid');
$intro = isset($_REQUEST['subject']) ? '[Mail] ' . trim($_REQUEST['subject']) : "You have a new message from {$from}";
$emailText = "";
foreach ($_POST as $key => $value) {
// If the field exists in the $fields array, include it in the email
if (isset($fields[$key])) {
if (empty($value)) continue;
$emailText .= "$fields[$key]: $value\n";
}
}
if (empty(trim($emailText))) throw new \Exception('Messages Empty');
$emailText = "$intro\n=============================\n\n{$emailText}\n=============================\n";
// All the neccessary headers for the email.
$headers = array(
'Content-Type: text/plain; charset="UTF-8";',
'From: ' . $from,
'Reply-To: ' . $from,
'Return-Path: ' . $from,
);
// Send email
mail($sendTo, $subject, $emailText, implode("\n", $headers));
$responseArray = array('type' => 'success', 'message' => $okMessage);
} catch (\Exception $e) {
$responseArray = array('type' => 'danger', 'message' => $errorMessage . '. ' . $e->getMessage());
}
$encoded = json_encode($responseArray);
echo $encoded;
<form class="p-5 grey-text" id="cForm">
<div class="md-form form-sm"> <i class="fa fa-user prefix"></i>
<input type="text" id="form3" name="name" class="form-control form-control-sm">
<label for="form3">Your name</label>
</div>
<div class="md-form form-sm"> <i class="fa fa-envelope prefix"></i>
<input type="text" id="form2" name="email" class="form-control form-control-sm">
<label for="form2">Your email</label>
</div>
<div class="md-form form-sm"> <i class="fa fa-tag prefix"></i>
<input type="text" id="form32" name="subject" class="form-control form-control-sm">
<label for="form34">Subject</label>
</div>
<div class="md-form form-sm"> <i class="fa fa-pencil-alt prefix"></i>
<textarea name="message" type="text" id="form8" class="md-textarea form-control form-control-sm"
rows="4"></textarea>
<label for="form8">Your message</label>
</div>
<div class="text-center mt-4">
<button class="btn btn-primary waves-effect waves-light">Send <i class="fa fa-paper-plane ml-1"></i></button>
</div>
</form>
Google SMTP
Send mail using google smtp with php
Requirement:- PHP PEAR MAIL or install using composer
or install using pear
composer require phpmailer/phpmailer
composer require pear/net_smtp
composer require pear/mail
pear install mail
html form
<?php
$subject = isset($_REQUEST['subject']) ? trim($_REQUEST['subject']) : 'Subject Mail';
$body = isset($_REQUEST['body']) ? trim($_REQUEST['body']) : 'Body Mail';
$from = isset($_REQUEST['from']) ? trim($_REQUEST['from']) : 'dimascyber008@gmail.com';
$body = "$body\n#end";
$headers = [
'From' => $from,
'To' => $to,
'Subject' => $subject,
'MIME-Version' => '1.0',
'Content-Type' => 'text/html; charset=UTF-8',
];
$smtp = Mail::factory('smtp', [
'host' => 'ssl://smtp.gmail.com',
'port' => '465',
'auth' => true,
'username' => 'dimascyber008@gmail.com',
'password' => 'oacjnzwovgejrdjb',
]);
$mail = $smtp->send($to, $headers, $body);
$result = [];
if (PEAR::isError($mail)) {
$result['error'] = true;
$result['message'] = $mail->getMessage();
$result['header'] = json_encode($headers);
} else {
try {
$result['success'] = true;
$result['message'] = 'Message successfully sent';
} catch (Exception $e) {
$result['error'] = true;
$result['message'] = $e->getMessage();
}
}
echo json_encode($result, JSON_PRETTY_PRINT | JSON_UNESCAPED_SLASHES | JSON_UNESCAPED_UNICODE);
?>
<form action="namefile.php" method="post">
<input type="email" name="from" placeholder="Your email" class="form-control">
<input type="text" name="subject" placeholder="Subject Mail" class="form-control">
<textarea name="body" id="" cols="30" rows="10" class="form-control" placeholder="your messages"></textarea>
</form>
Incoming terms:
- php mail ajax
- send mail using php
- easy send mail with php
- php mail with ajax
- php mail using ajax
- how to send mail with ajax
- how to send mail using ajax
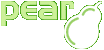
